Tic-Tac-Toe: Look for Wins
In earlier labs, you worked out how to display moves on a tic-tac-toe board and keep track of them in a variable board whose value is a list of nine values, one per square of the board, each of which can be X or O or empty if that square is unoccupied:
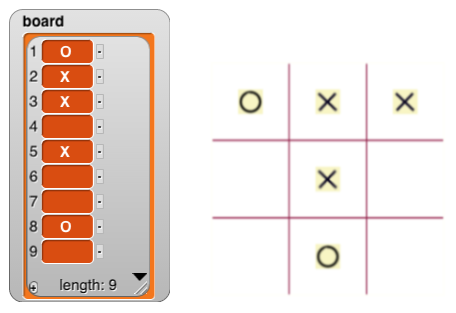
Now we'd like the computer to be a player in the game, and to do that, it has to be able to detect patterns in the board position that mean, for example, "I can win on the next move."
- How many ways are there to win at tic-tac-toe? That is, which sets of three squares count as a win? Work with your partner to make a list on paper.
- Load this project. We've provided a
wins
block that reports a list of all the winning triples:
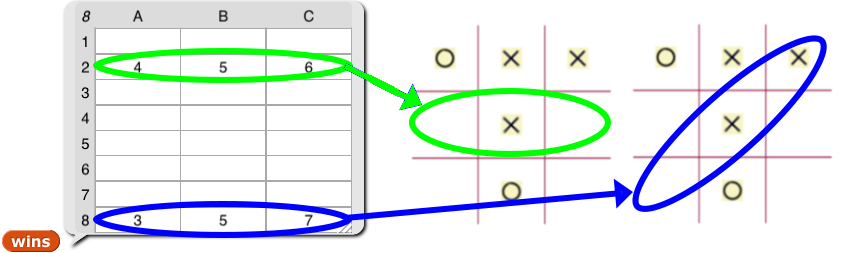
- Try these:
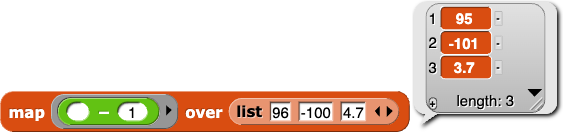
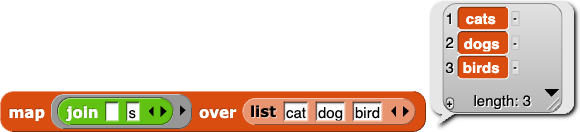
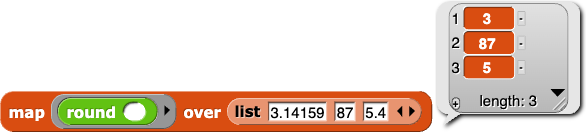
Note that each example has an empty input slot in a function (the green blocks, in these examples).
Read this block definition:

- If triple is
{4,5,6}
and board is as shown above, what will the value of occupied be after the set
block is run?
- The
report
block says to report

Say in words what would make sense as the something
s: two of what, and zero of what, are required for a winning triple?
- Looking at the actual code, figure out what the
keep
block does. Here's a simpler example to consider:

- Write a block
that reports a list of all winning triples for that player, using the list reported by wins
.
- If the computer can win on this move, it should. Otherwise, if the opponent can win on this move, the computer should block that. But if neither of those is true, at least the computer should find a place to move in a triple where it already appears once, and the opponent doesn't appear, such as
{4,5,6}
for X on this board. Write 
- Actually, on this board, it's not X's turn; it's O's turn. There is no winning move for O according to the block you wrote in problem 7, but there is one great square for O to choose. Which is it?
- Write a procedure to find such a move.